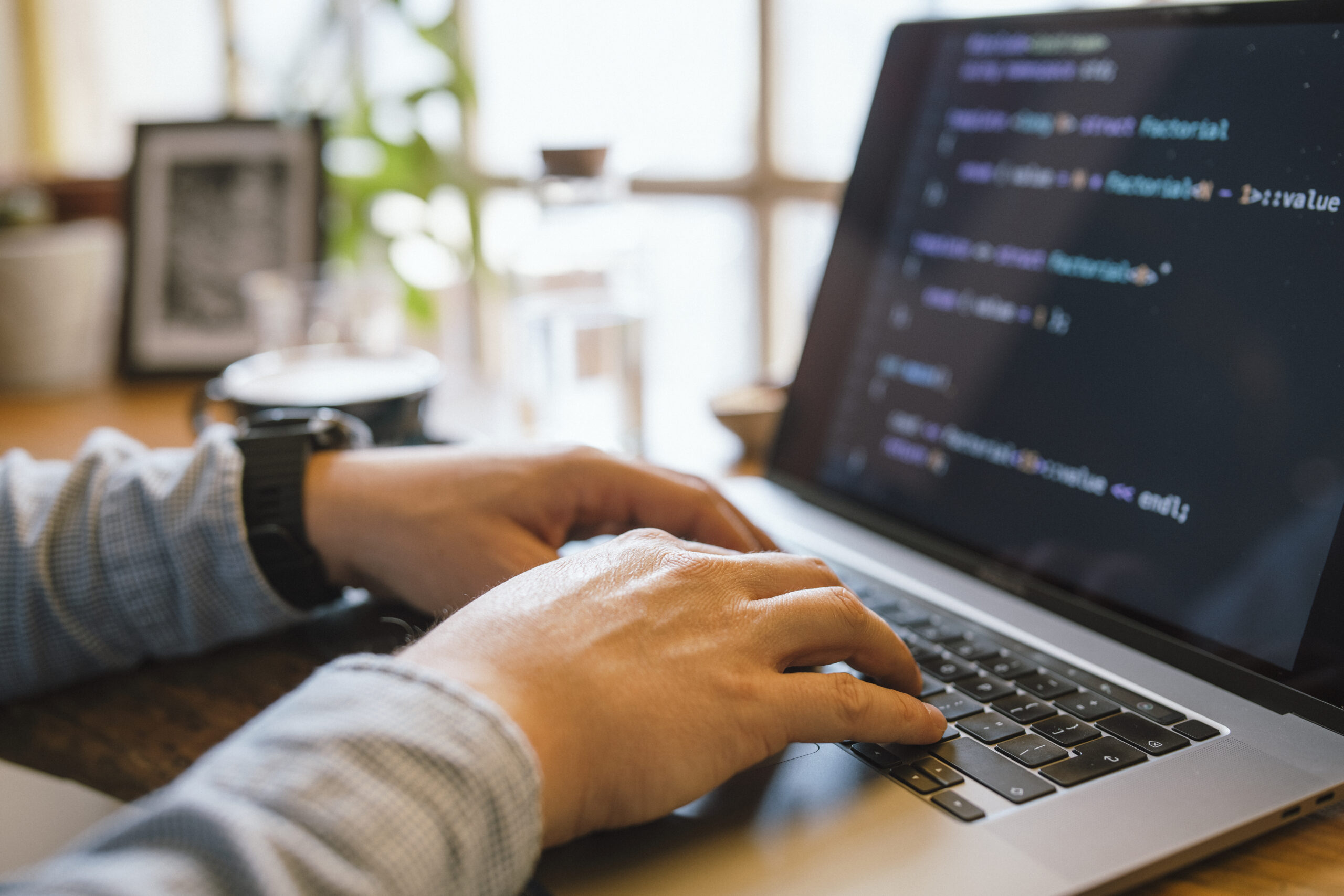
Debugging is Probably the most crucial — but generally missed — abilities inside a developer’s toolkit. It is not almost fixing broken code; it’s about comprehending how and why items go Improper, and Finding out to Consider methodically to unravel complications competently. Whether or not you're a beginner or a seasoned developer, sharpening your debugging skills can conserve hrs of disappointment and substantially boost your productivity. Listed here are many approaches to aid developers level up their debugging activity by me, Gustavo Woltmann.
Learn Your Applications
On the list of fastest techniques developers can elevate their debugging skills is by mastering the applications they use every day. Though producing code is one particular Portion of development, recognizing how to connect with it proficiently for the duration of execution is equally vital. Modern-day advancement environments arrive equipped with highly effective debugging capabilities — but many builders only scratch the surface of what these tools can perform.
Get, for example, an Built-in Development Natural environment (IDE) like Visual Studio Code, IntelliJ, or Eclipse. These resources assist you to set breakpoints, inspect the worth of variables at runtime, stage as a result of code line by line, and in some cases modify code within the fly. When used effectively, they let you notice exactly how your code behaves for the duration of execution, that's a must have for tracking down elusive bugs.
Browser developer instruments, like Chrome DevTools, are indispensable for entrance-end developers. They permit you to inspect the DOM, watch network requests, check out serious-time overall performance metrics, and debug JavaScript from the browser. Mastering the console, sources, and community tabs can turn aggravating UI challenges into manageable duties.
For backend or process-stage builders, tools like GDB (GNU Debugger), Valgrind, or LLDB supply deep Regulate over working procedures and memory management. Mastering these applications might have a steeper Understanding curve but pays off when debugging general performance difficulties, memory leaks, or segmentation faults.
Over and above your IDE or debugger, develop into snug with version Manage programs like Git to be aware of code record, uncover the precise minute bugs were being released, and isolate problematic changes.
In the end, mastering your resources usually means going beyond default settings and shortcuts — it’s about creating an intimate understanding of your progress ecosystem so that when issues arise, you’re not lost in the dark. The better you recognize your equipment, the more time you'll be able to devote solving the actual problem as opposed to fumbling by means of the process.
Reproduce the issue
Probably the most crucial — and often missed — measures in efficient debugging is reproducing the issue. Before leaping in the code or producing guesses, developers require to create a dependable natural environment or circumstance in which the bug reliably seems. Devoid of reproducibility, correcting a bug gets a recreation of chance, normally resulting in wasted time and fragile code variations.
The initial step in reproducing a difficulty is gathering just as much context as you possibly can. Ask issues like: What actions brought about the issue? Which natural environment was it in — advancement, staging, or production? Are there any logs, screenshots, or mistake messages? The more depth you might have, the simpler it results in being to isolate the exact disorders beneath which the bug takes place.
As soon as you’ve gathered more than enough details, try to recreate the challenge in your local setting. This may suggest inputting exactly the same facts, simulating comparable consumer interactions, or mimicking system states. If The problem seems intermittently, contemplate composing automatic tests that replicate the edge conditions or state transitions included. These checks not merely assistance expose the trouble and also prevent regressions Later on.
From time to time, the issue could be atmosphere-distinct — it'd happen only on particular running devices, browsers, or under unique configurations. Applying tools like virtual devices, containerization (e.g., Docker), or cross-browser screening platforms is often instrumental in replicating such bugs.
Reproducing the trouble isn’t just a step — it’s a attitude. It calls for endurance, observation, in addition to a methodical solution. But once you can continually recreate the bug, you might be already midway to correcting it. With a reproducible scenario, You should use your debugging resources additional proficiently, exam opportunity fixes properly, and connect extra Evidently with all your team or customers. It turns an abstract criticism right into a concrete problem — and that’s the place developers prosper.
Examine and Recognize the Error Messages
Error messages are often the most valuable clues a developer has when something goes Improper. Instead of seeing them as frustrating interruptions, builders need to find out to treat mistake messages as immediate communications with the technique. They usually tell you exactly what took place, the place it happened, and at times even why it happened — if you know the way to interpret them.
Commence by studying the information meticulously and in full. Numerous builders, particularly when less than time force, glance at the main line and quickly begin creating assumptions. But further inside the mistake stack or logs may possibly lie the true root bring about. Don’t just copy and paste mistake messages into serps — go through and understand them 1st.
Break the error down into pieces. Could it be a syntax mistake, a runtime exception, or even a logic error? Will it point to a particular file and line selection? What module or operate brought on it? These inquiries can guide your investigation and issue you toward the dependable code.
It’s also helpful to grasp the terminology of the programming language or framework you’re making use of. Mistake messages in languages like Python, JavaScript, or Java often abide by predictable patterns, and Finding out to acknowledge these can significantly hasten your debugging system.
Some mistakes are obscure or generic, As well as in Individuals scenarios, it’s crucial to examine the context through which the mistake happened. Verify relevant log entries, input values, and recent adjustments from the codebase.
Don’t ignore compiler or linter warnings either. These usually precede more substantial issues and provide hints about probable bugs.
Finally, mistake messages aren't your enemies—they’re your guides. Understanding to interpret them accurately turns chaos into clarity, serving to you pinpoint challenges faster, lessen debugging time, and turn into a additional economical and self-assured developer.
Use Logging Sensibly
Logging is one of the most potent resources within a developer’s debugging toolkit. When made use of correctly, it offers serious-time insights into how an software behaves, serving to you have an understanding of what’s going on underneath the hood while not having to pause execution or move in the code line by line.
A very good logging system starts off with figuring out what to log and at what stage. Prevalent logging degrees include things like DEBUG, Facts, Alert, ERROR, and FATAL. Use DEBUG for detailed diagnostic information all through enhancement, Details for standard gatherings (like profitable commence-ups), WARN for potential challenges that don’t split the application, Mistake for genuine challenges, and Deadly when the system can’t continue on.
Keep away from flooding your logs with extreme or irrelevant data. An excessive amount logging can obscure critical messages and decelerate your method. Focus on critical activities, state improvements, input/output values, and significant selection factors inside your code.
Structure your log messages Obviously and continuously. Incorporate context, like timestamps, ask for IDs, and function names, so it’s simpler to trace troubles in distributed programs or multi-threaded environments. Structured logging (e.g., JSON logs) could make it even simpler to parse and filter logs programmatically.
Throughout debugging, logs Permit you to track how variables evolve, what ailments are met, and what branches of logic are executed—all devoid of halting This system. They’re especially worthwhile in production environments in which stepping as a result of code isn’t achievable.
On top of that, use logging frameworks and resources (like Log4j, Winston, or Python’s logging module) that guidance log rotation, filtering, and integration with monitoring dashboards.
Finally, wise logging is about harmony and clarity. Which has a effectively-assumed-out logging strategy, you may reduce the time it requires to identify problems, achieve further visibility into your applications, and improve the Total maintainability and trustworthiness of your code.
Feel Just like a Detective
Debugging is not just a specialized undertaking—it is a form of investigation. To successfully discover and deal with bugs, builders must method the procedure similar to a detective resolving a mystery. This state of mind aids break down intricate challenges into workable parts and stick to clues logically to uncover the basis bring about.
Start out by accumulating proof. Think about the symptoms of the challenge: error messages, incorrect output, or overall performance issues. Just like a detective surveys a crime scene, collect just as much relevant info as you are able to without having jumping to conclusions. Use logs, examination conditions, and person stories to piece jointly a transparent image of what’s taking place.
Following, kind hypotheses. Request oneself: What may very well be resulting in this habits? Have any improvements not long ago been manufactured on the codebase? Has this concern occurred before less than similar instances? The target is usually to narrow down possibilities and detect probable culprits.
Then, examination your theories systematically. Make an effort to recreate the issue inside of a controlled ecosystem. When you suspect a certain perform or ingredient, isolate it and confirm if The difficulty persists. Just like a detective conducting interviews, inquire your code thoughts and Permit the final results direct you nearer to the reality.
Pay out close awareness to tiny details. Bugs generally conceal within the the very least anticipated sites—just like a lacking semicolon, an off-by-one particular mistake, or even a race condition. Be extensive and affected Gustavo Woltmann AI person, resisting the urge to patch The difficulty with no fully knowledge it. Temporary fixes may possibly hide the true problem, only for it to resurface afterwards.
Finally, retain notes on That which you tried and uncovered. Equally as detectives log their investigations, documenting your debugging method can help you save time for long term difficulties and help Other folks have an understanding of your reasoning.
By considering similar to a detective, developers can sharpen their analytical expertise, tactic issues methodically, and turn into more practical at uncovering concealed problems in advanced systems.
Create Checks
Creating assessments is among the most effective methods to increase your debugging competencies and General advancement effectiveness. Assessments don't just aid capture bugs early and also function a security Web that offers you self-confidence when creating adjustments to the codebase. A very well-analyzed application is easier to debug because it allows you to pinpoint precisely in which and when an issue occurs.
Start with unit tests, which concentrate on person features or modules. These modest, isolated exams can swiftly reveal regardless of whether a specific piece of logic is Doing the job as envisioned. Every time a take a look at fails, you quickly know where by to glance, appreciably minimizing time invested debugging. Unit checks are In particular valuable for catching regression bugs—concerns that reappear following previously being preset.
Upcoming, integrate integration tests and close-to-conclude exams into your workflow. These assist ensure that several areas of your application do the job together efficiently. They’re specifically useful for catching bugs that come about in intricate methods with various elements or services interacting. If a thing breaks, your exams can show you which Portion of the pipeline failed and less than what problems.
Writing assessments also forces you to Consider critically about your code. To check a feature appropriately, you'll need to be aware of its inputs, expected outputs, and edge cases. This standard of understanding Obviously leads to higher code composition and fewer bugs.
When debugging a concern, writing a failing examination that reproduces the bug is usually a powerful initial step. Once the examination fails continuously, you'll be able to center on fixing the bug and observe your take a look at pass when The problem is solved. This solution ensures that precisely the same bug doesn’t return Down the road.
In a nutshell, crafting tests turns debugging from a annoying guessing video game right into a structured and predictable process—aiding you capture additional bugs, faster and much more reliably.
Just take Breaks
When debugging a difficult issue, it’s simple to become immersed in the challenge—observing your monitor for several hours, trying Answer right after Resolution. But Among the most underrated debugging instruments is solely stepping absent. Getting breaks helps you reset your mind, decrease aggravation, and often see the issue from a new perspective.
When you're too close to the code for too long, cognitive exhaustion sets in. You might commence overlooking apparent mistakes or misreading code that you simply wrote just hours earlier. In this point out, your Mind will become a lot less successful at dilemma-fixing. A short wander, a espresso crack, as well as switching to a distinct activity for 10–quarter-hour can refresh your concentration. A lot of developers report finding the foundation of a challenge once they've taken time to disconnect, permitting their subconscious get the job done during the qualifications.
Breaks also aid stop burnout, especially through more time debugging sessions. Sitting down in front of a screen, mentally trapped, is not just unproductive but also draining. Stepping absent permits you to return with renewed Vitality and a clearer way of thinking. You could suddenly detect a missing semicolon, a logic flaw, or simply a misplaced variable that eluded you ahead of.
If you’re caught, a great general guideline is always to established a timer—debug actively for 45–sixty minutes, then have a five–10 moment crack. Use that time to maneuver about, extend, or do one thing unrelated to code. It may well really feel counterintuitive, Primarily below limited deadlines, however it essentially leads to a lot quicker and more effective debugging In the long term.
In brief, getting breaks is not a sign of weak point—it’s a sensible technique. It offers your Mind space to breathe, enhances your point of view, and helps you stay away from the tunnel eyesight that often blocks your progress. Debugging is often a psychological puzzle, and rest is a component of fixing it.
Master From Every Bug
Every single bug you come upon is more than just A brief setback—It can be a possibility to grow like a developer. No matter if it’s a syntax error, a logic flaw, or even a deep architectural challenge, every one can instruct you some thing useful in case you go to the trouble to replicate and analyze what went Incorrect.
Commence by asking by yourself some vital thoughts once the bug is settled: What triggered it? Why did it go unnoticed? Could it are already caught previously with greater techniques like device screening, code opinions, or logging? The responses generally expose blind spots within your workflow or comprehension and allow you to Create more robust coding behavior transferring ahead.
Documenting bugs can be a fantastic routine. Preserve a developer journal or sustain a log where you note down bugs you’ve encountered, the way you solved them, and That which you uncovered. After a while, you’ll start to see patterns—recurring challenges or prevalent problems—which you can proactively stay away from.
In crew environments, sharing Everything you've learned from the bug using your peers can be Primarily highly effective. No matter whether it’s through a Slack information, a short write-up, or A fast information-sharing session, helping Many others stay away from the same difficulty boosts crew effectiveness and cultivates a stronger Mastering tradition.
Extra importantly, viewing bugs as lessons shifts your mindset from annoyance to curiosity. As opposed to dreading bugs, you’ll get started appreciating them as vital parts of your growth journey. In the end, a lot of the greatest builders usually are not those who create great code, but individuals who continuously understand from their mistakes.
Ultimately, Each individual bug you resolve provides a brand new layer towards your skill set. So future time you squash a bug, take a second to replicate—you’ll come away a smarter, additional able developer as a result of it.
Summary
Improving upon your debugging expertise requires time, follow, and tolerance — but the payoff is big. It would make you a far more efficient, assured, and able developer. The subsequent time you might be knee-deep inside a mysterious bug, don't forget: debugging isn’t a chore — it’s a possibility to be better at Everything you do.